In this article, we will delve into automating the creation of Jira issues using Python and the Jira REST API. Jira is a powerful tool for project management and issue tracking, and automating repetitive tasks such as issue creation can significantly enhance productivity and efficiency in software development teams.
Jira provides a robust REST API that allows developers to interact with Jira programmatically. This API can be utilized to perform various operations, including creating, updating, and retrieving issues, managing projects, and more. Automating Jira issue creation using Python enables teams to streamline their workflow, reduce manual effort, and ensure consistent data entry.
Table of Contents
Prerequisites
- AWS Account with Ubuntu 24.04 LTS EC2 Instance.
- A Jira account with a project set.
- Basic knowledge of python.
Step #1:Create a Jira Project
Log in into Jira account.
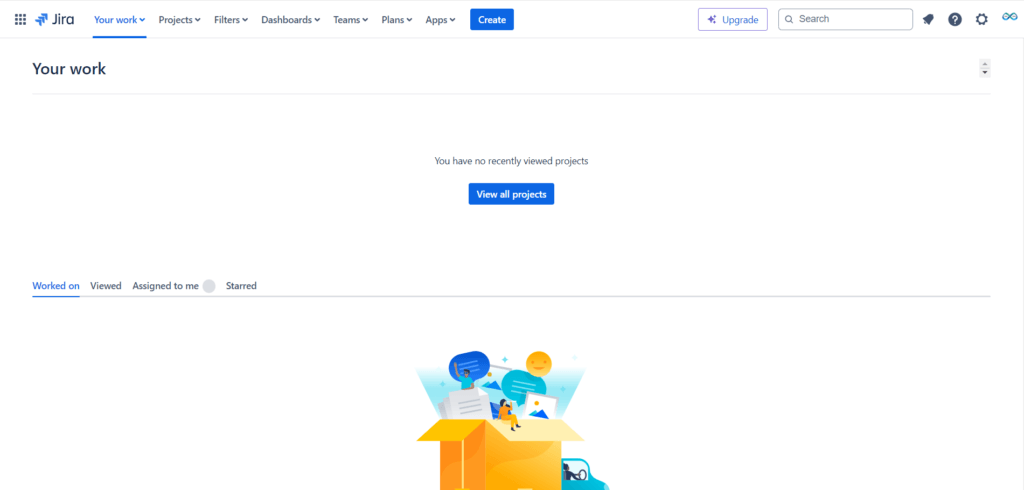
After logging in, create a new project by selecting the Create project from Projects.
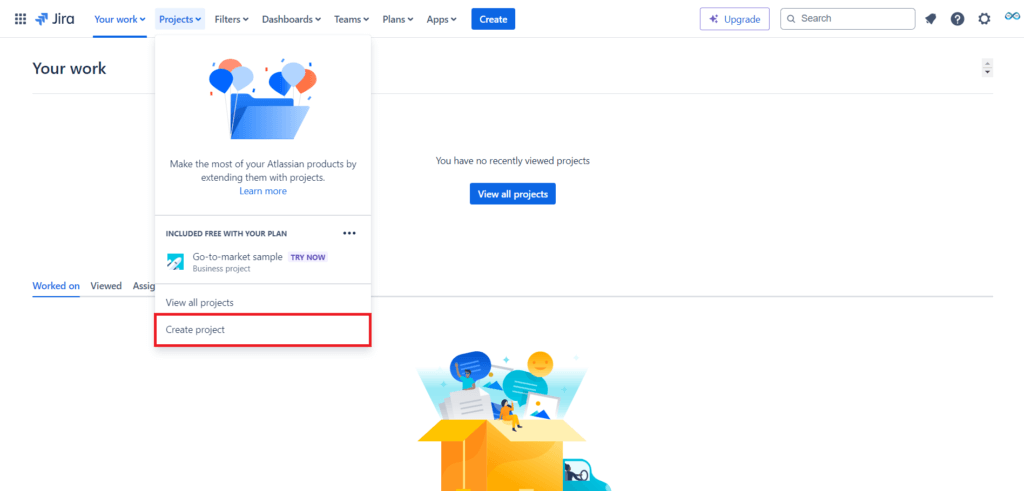
select the Scrum template from Software development for simplicity.
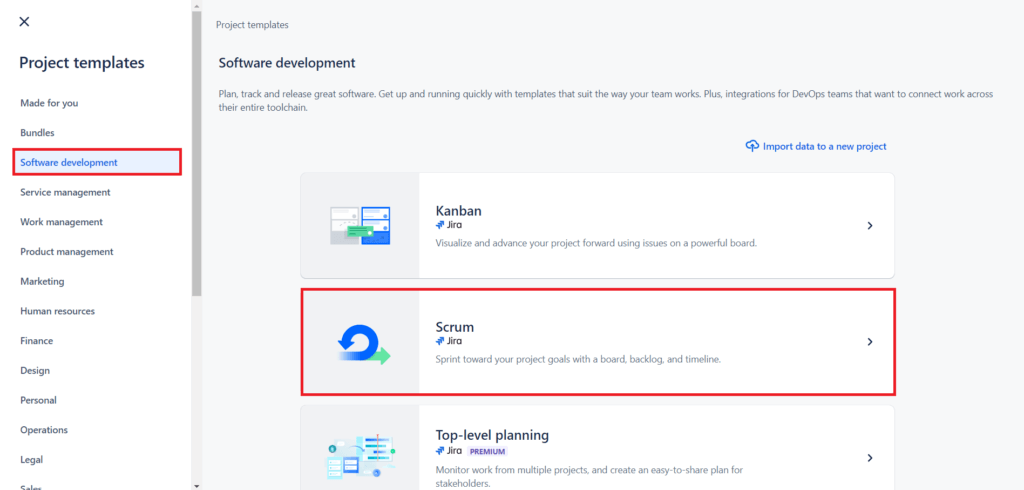
Click on Use template.
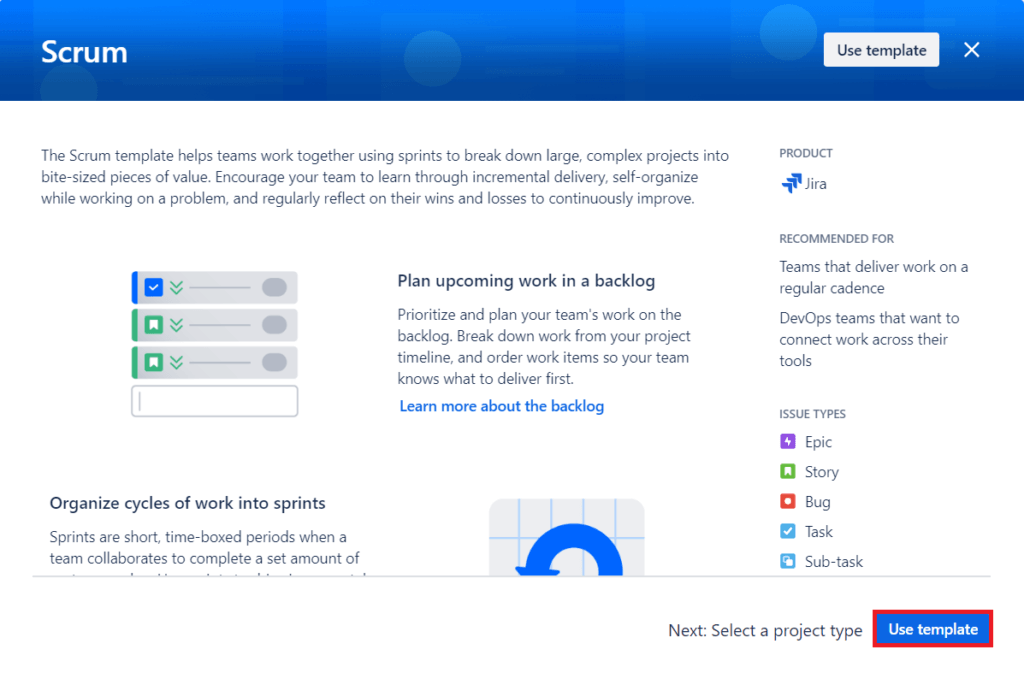
Choose a project type from team-managed project and company-managed project.
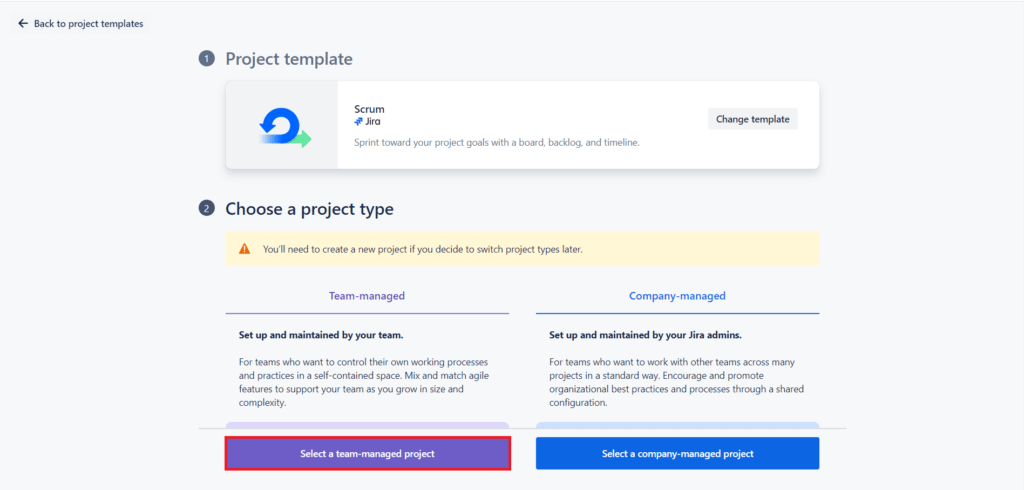
Add the project details. Provide a project name and key is generated. Click on Next.
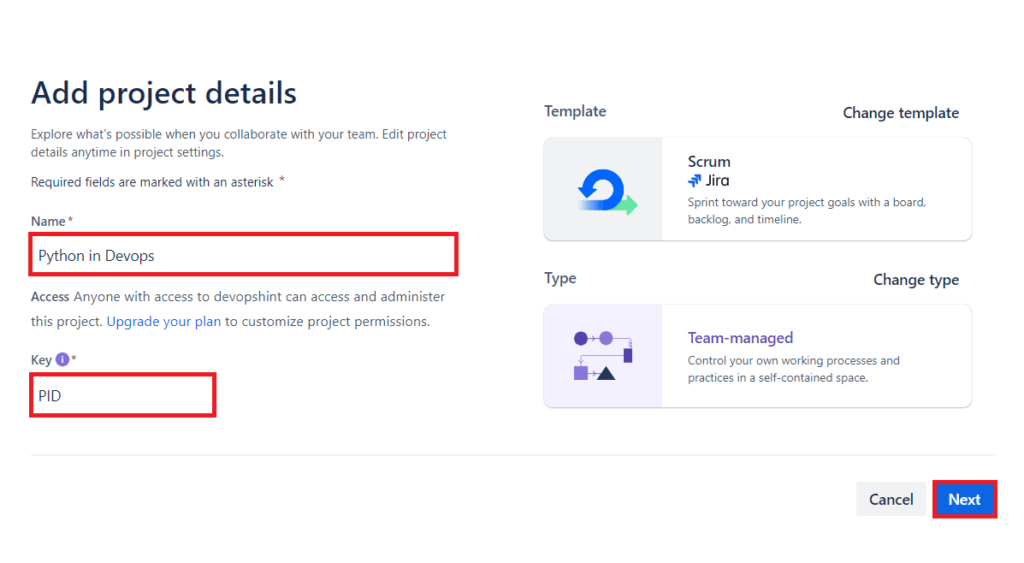
Project is created successfully.
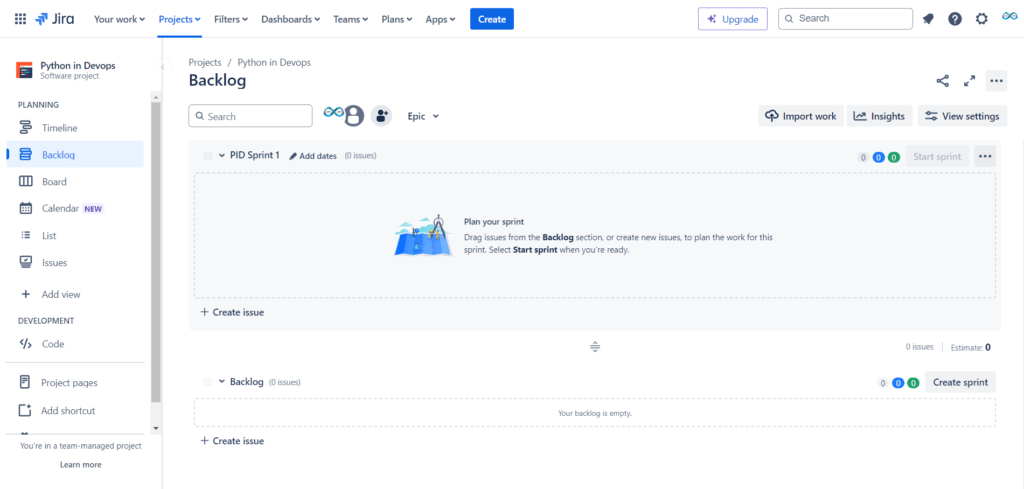
Step #2:Generate API Token
Go to your Jira profile.

Click on Manage your account.

Under Security, select Create and manage API token.
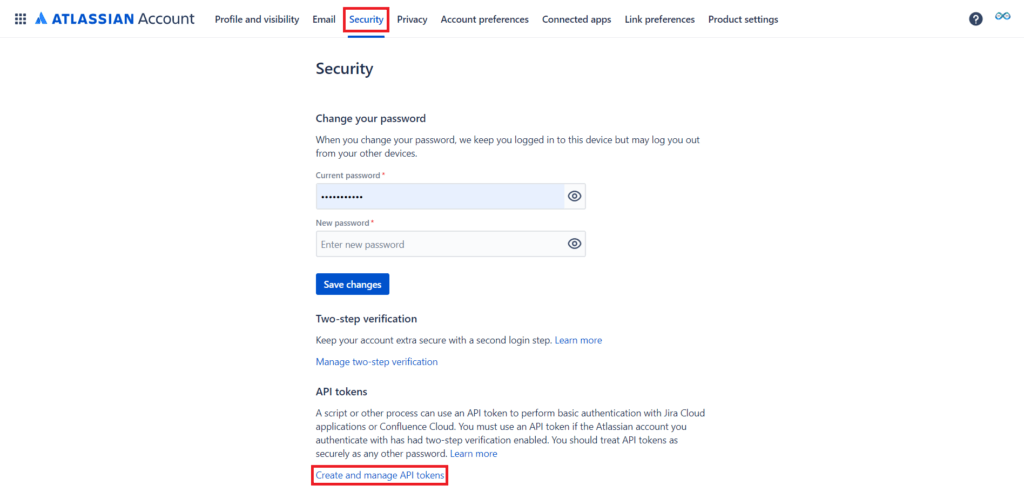
Click on Create API token.
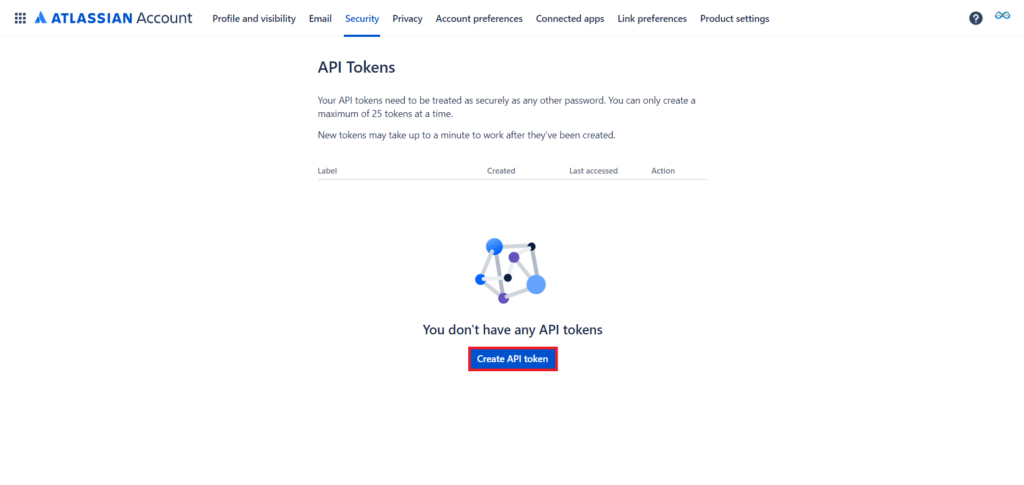
Provide a label and click on Create.
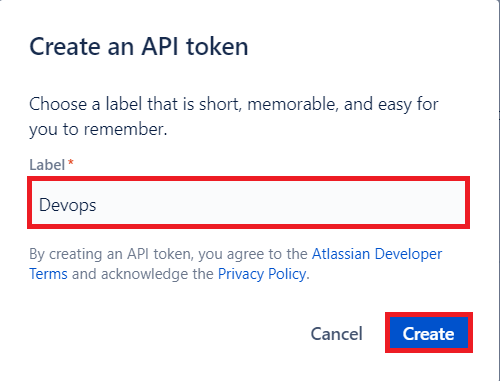
Copy the generated API token cause we will be using it later.

Now our API token is also created.

Step #3:Install Python and Required Tools
First update the system packages.
sudo apt update

Install Python, Python Virtual Environment and Pip.
sudo apt install python3 python3-venv python3-pip -y

Set up a Python Virtual Environment. A virtual environment isolates your Python environment from the global system environment, making dependency management easier and avoiding conflicts
python3 -m venv myenv

Activate the virtual environment to use it.
source myenv/bin/activate

With the virtual environment active, use Pip to install the requests library, which we need for making HTTP requests to the Jira API.
pip install requests

Step #4:Write a python script to retrieve a list of all projects in Jira
First create a directory and navigate to it.
mkdir ~/jira_integration
cd ~/jira_integration

Create a file to write python script like main.py
nano main.py

add the code into it.
import requests
from requests.auth import HTTPBasicAuth
import json
url = "https://devopshint.atlassian.net/rest/api/3/project"
auth = HTTPBasicAuth("[email protected]", "ATATT3xFfGF0zktRyCVLhR9VVenbufEtiYTtCiJBfPvR9y3DfKBtffFewvsFhgoUj04BPiJbV_TZMToxbn8WeANEZwoZf1g5AV8RmKIFSY8YPS_wx1zhi7u-YXAX0zAB15cpYHnIoC_NuguFBfos2-4NlI7bbsEzvxnCZy3WTg612KI-WVHCSOI=3837B89F")
headers = {
"Accept": "application/json"
}
response = requests.request(
"GET",
url,
headers=headers,
auth=auth
)
print(json.dumps(json.loads(response.text), sort_keys=True, indent=4, separators=(",", ": ")))
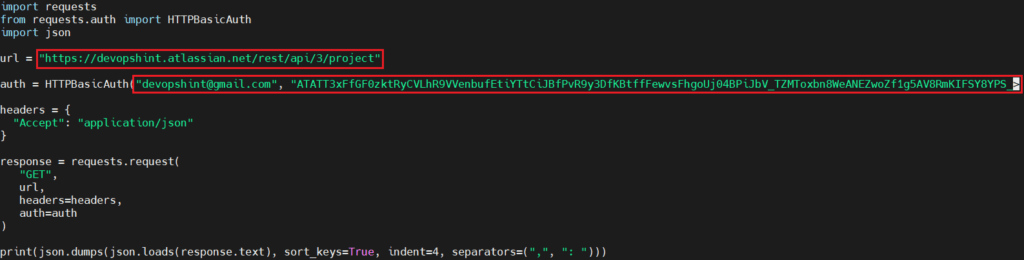
Give your own domain in url, email and API token. Save the file and exit.
Explanation of the script:
- Import Required Libraries:
requests
: A Python library for sending HTTP requests.HTTPBasicAuth
: A utility from therequests.auth
module to handle HTTP Basic Authentication.json
: A library for working with JSON data.
- Set the API Endpoint URL:
url
: The API endpoint for fetching project information. Replace"https://your-domain.atlassian.net"
with your actual Jira domain.
- Authentication:
auth
: AnHTTPBasicAuth
object created with the Jira user’s email and an API token. Replace"[email protected]"
and"<api_token>"
with your actual email and API token. The API token can be generated from your Jira profile under the API tokens section.
- Set Request Headers:
headers
: A dictionary specifying that the response should be in JSON format."Accept": "application/json"
indicates that the client expects a JSON response.
- Send the HTTP GET Request:
- The script uses
requests.request
to send aGET
request to the Jira API. It includes theurl
,headers
, andauth
for authentication.
- The script uses
- Process and Print the Response:
response.text
: Contains the raw JSON response from the server.json.loads(response.text)
: Parses the JSON response into a Python dictionary.json.dumps(..., sort_keys=True, indent=4, separators=(",", ": "))
: Converts the dictionary back to a formatted JSON string for easy reading and prints it.
Run the script. The output is formatted JSON string containing details about all projects accessible to the authenticated user.
python main.py

Step #5:Create Issue in Jira
Now we want to create an issue in Jira. So lets create a file to write a python script to create issue.
nano issue.py

To get the issue id to write in script go to your project>> configure board >> the issue you want to create, see url you will get issue id
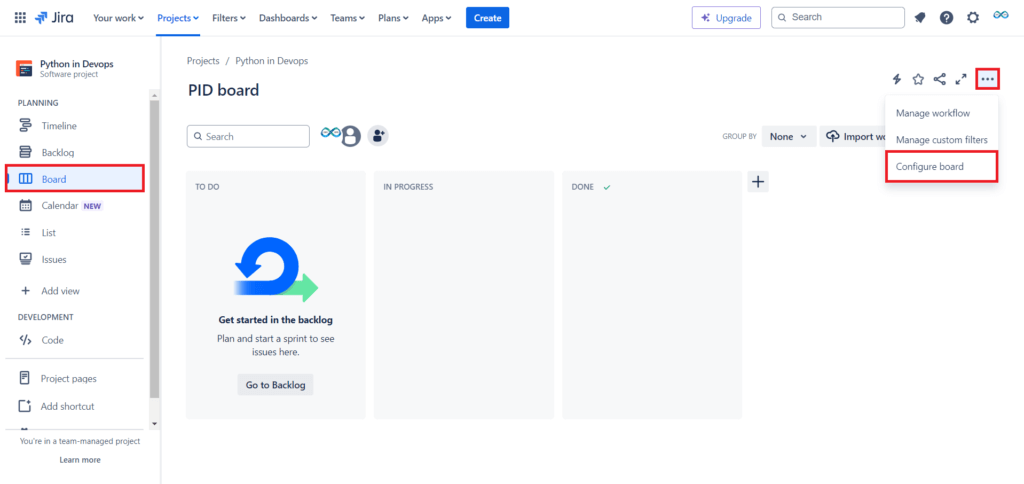

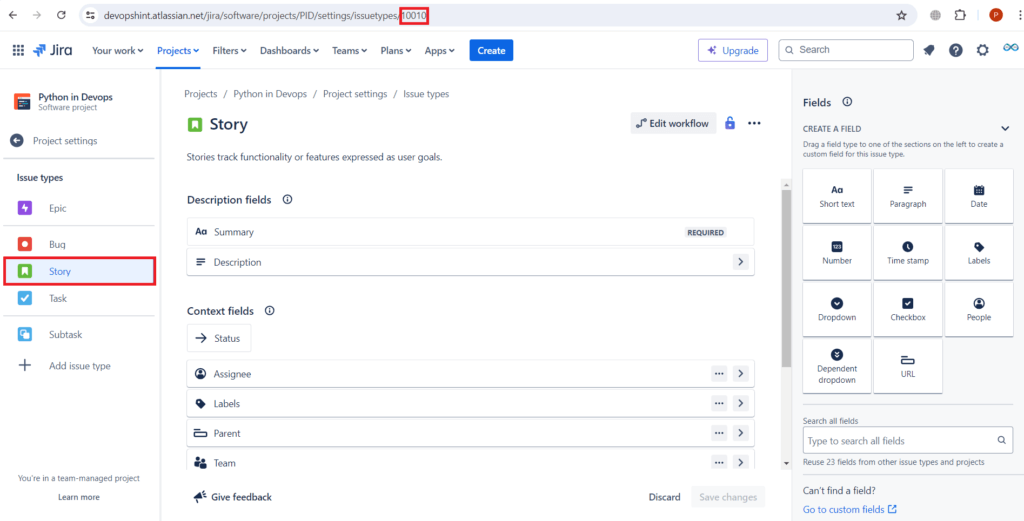
import requests
from requests.auth import HTTPBasicAuth
import json
url = "https://your_domain.atlassian.net/rest/api/3/issue"
auth = HTTPBasicAuth("[email protected]", "YOUR_API_TOKEN")
headers = {
"Accept": "application/json",
"Content-Type": "application/json"
}
payload = json.dumps( {
"fields": {
"description": {
"content": [
{
"content": [
{
"text": "My first Jira ticket of the day",
"type": "text"
}
],
"type": "paragraph"
}
],
"type": "doc",
"version": 1
},
"issuetype": {
"id": "YOUR_ID"
},
"project": {
"key": "YOUR_KEY"
},
"summary": "Main order flow broken",
},
"update": {}
} )
response = requests.request(
"POST",
url,
data=payload,
headers=headers,
auth=auth
)
print(json.dumps(json.loads(response.text), sort_keys=True, indent=4, separators=(",", ": ")))

Give your own domain in url, email and API token. Also give your id and key as shown in above image. Save the file and exit.
Explanation of the script:
- Import Required Libraries:
requests
: A Python library for sending HTTP requests.HTTPBasicAuth
: A utility from therequests.auth
module to handle HTTP Basic Authentication.json
: A library for working with JSON data.
- Set the API Endpoint URL:
url
: The Jira API endpoint for creating a new issue. Replace"https://devopshint.atlassian.net"
with your actual Jira domain.
- Authentication:
auth
: AnHTTPBasicAuth
object created with your Jira user’s email and an API token. Replace"[email protected]"
and"your_api_token_here"
with your actual email and API token. The API token is necessary to authenticate and interact with the Jira API securely.
- Set Request Headers:
headers
: A dictionary specifying that the request and response should be in JSON format.
- Prepare the Payload:
payload
: This is the JSON data containing the details of the issue to be created. It specifies:description
: A rich-text format description of the issue.issuetype
: The ID of the issue type (e.g., bug, task, etc.). Replace"10010"
with the correct issue type ID for your project.project
: The key of the project where the issue will be created. Replace"PID"
with the actual project key.summary
: A brief summary of the issue.
- Send the HTTP POST Request:
requests.request
: This sends aPOST
request to the Jira API endpoint with the payload and headers for creating the issue.
- Process and Print the Response:
response.text
: Contains the raw JSON response from the server.json.loads(response.text)
: Parses the JSON response into a Python dictionary.json.dumps(..., sort_keys=True, indent=4, separators=(",", ": "))
: Converts the dictionary back to a formatted JSON string for easy reading and prints it.
Run the script. The output is a formatted JSON string containing details about the created issue.
python issue.py

Now go to Jira and open the project backlog. You can see the created issue.
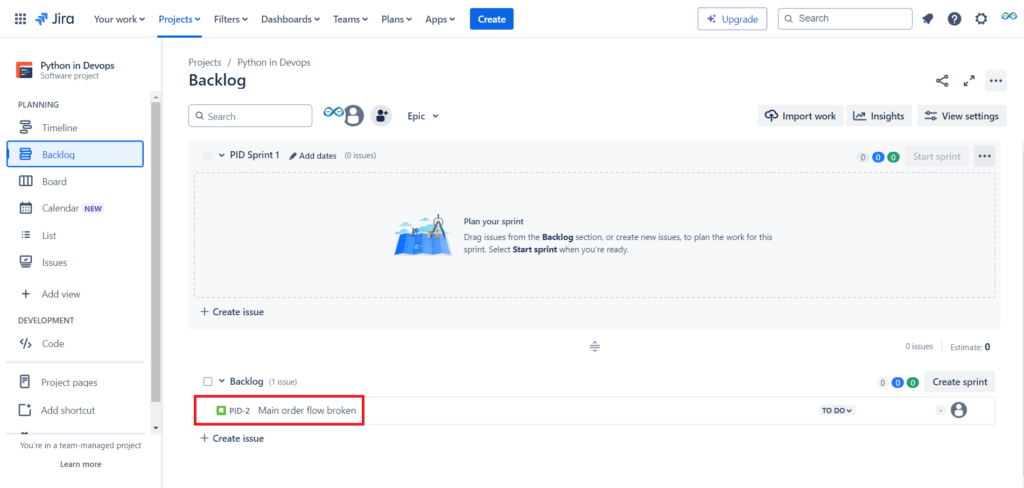
Conclusion:
In conclusion, the Jira REST API plays a crucial role in enabling developers and teams to interact programmatically with Jira, automating workflows, integrating with other systems, and enhancing productivity in software development and project management processes. Understanding and leveraging the capabilities of the Jira REST API empowers teams to streamline their operations and focus more on delivering high-quality software solutions.
Related Articles:
Shell Script to Monitor Disk Space Utilization and Send Email Alerts
Reference: