In this article, we will learn how to automate the creation of Jira tickets directly from GitHub comments using a Python script. This solution aims to streamline the process of issue tracking and management by eliminating the need for developers to manually log in to Jira and create tickets. By leveraging GitHub webhooks and the Jira REST API, we can automate ticket creation based on specific comments made on GitHub issues, thereby enhancing productivity and ensuring that important issues are tracked systematically.
Table of Contents
Prerequisites
- AWS Account with Ubuntu 24.04 LTS EC2 Instance.
- A Jira account with a project set.
- Basic knowledge of python.
Step #1:Create a Jira Project
Log in into Jira account.
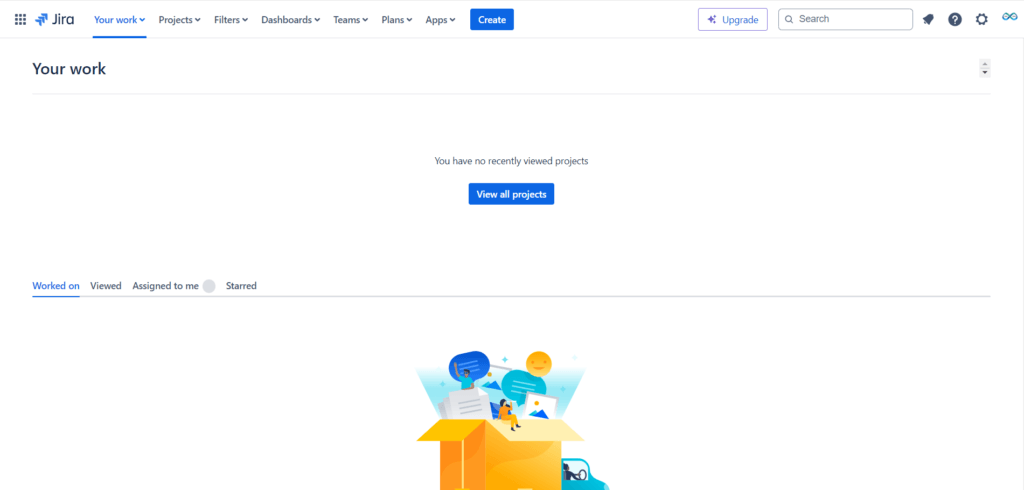
After logging in, create a new project by selecting the Create project from Projects.
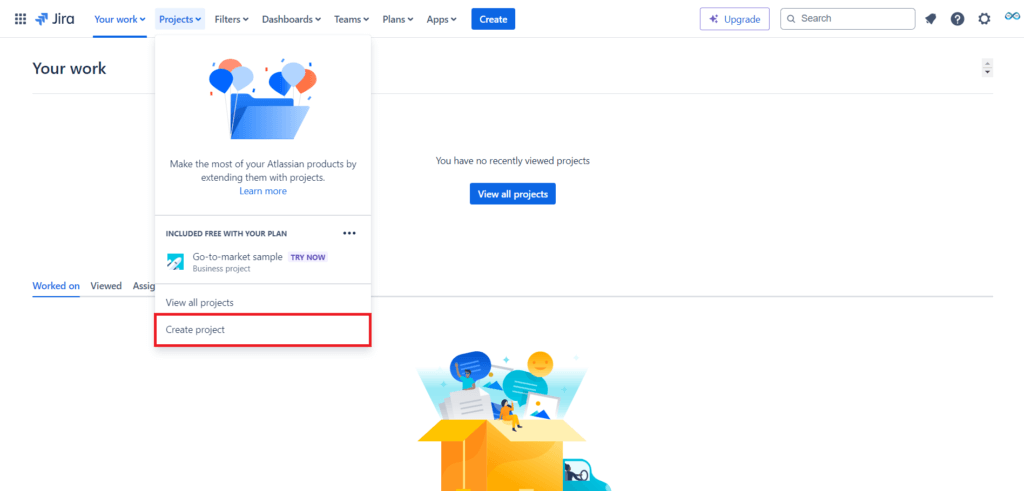
select the Scrum template from Software development for simplicity.
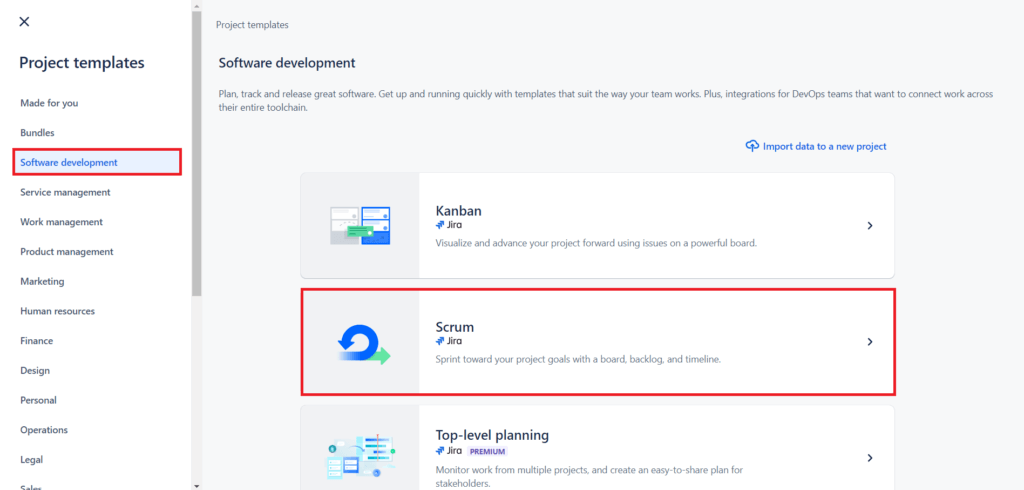
Click on Use template.
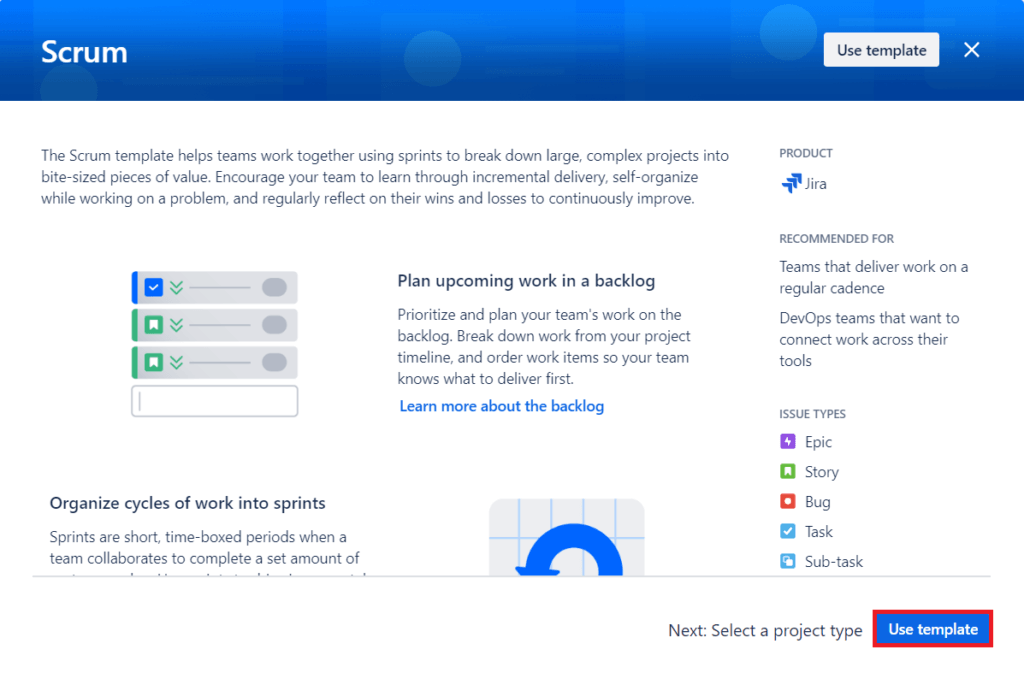
Choose a project type from team-managed project and company-managed project.
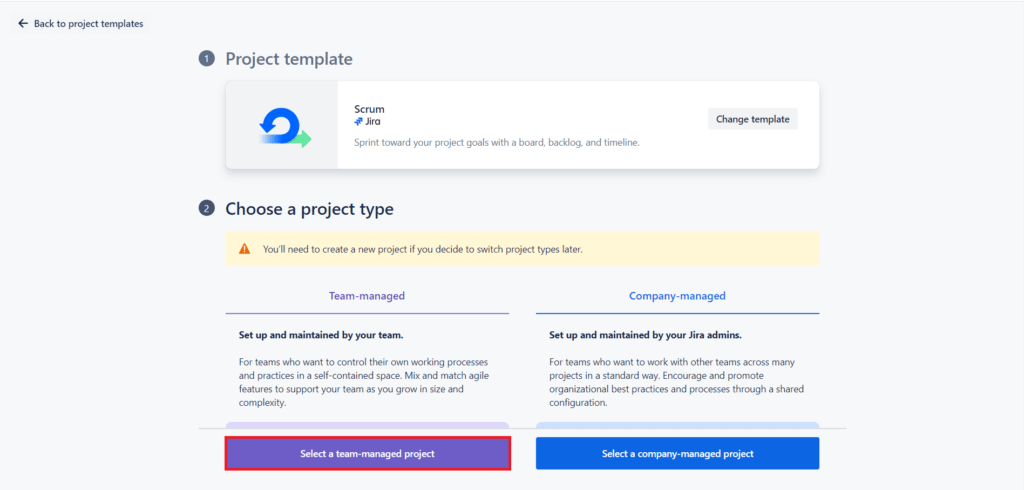
Add the project details. Provide a project name and key is generated. Click on Next.
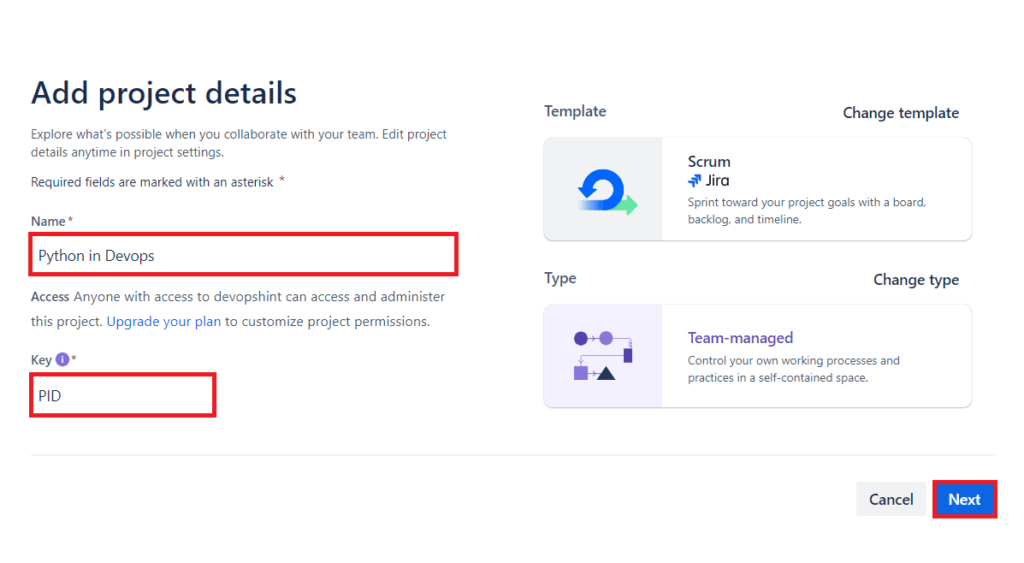
Project is created successfully.
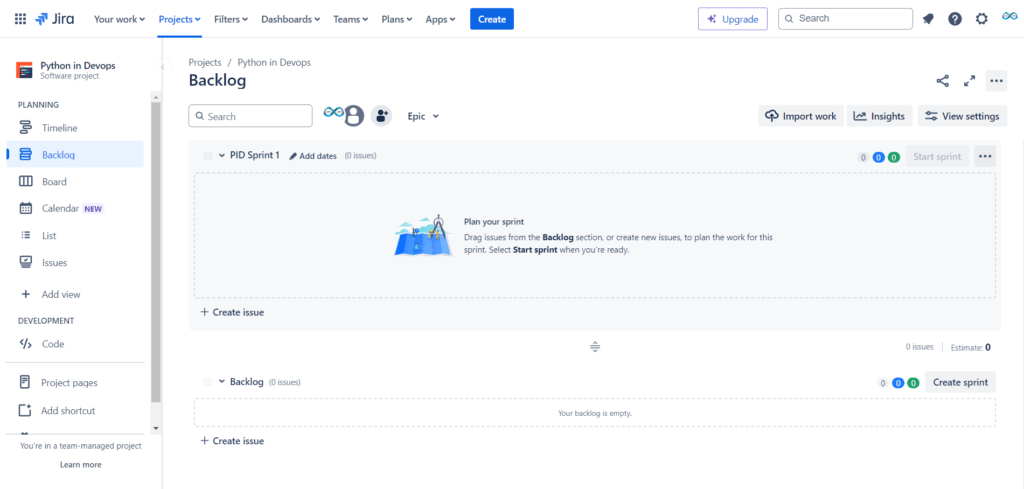
Step #2:Generate API Token
Go to your Jira profile.

Click on Manage your account.

Under Security, select Create and manage API token.
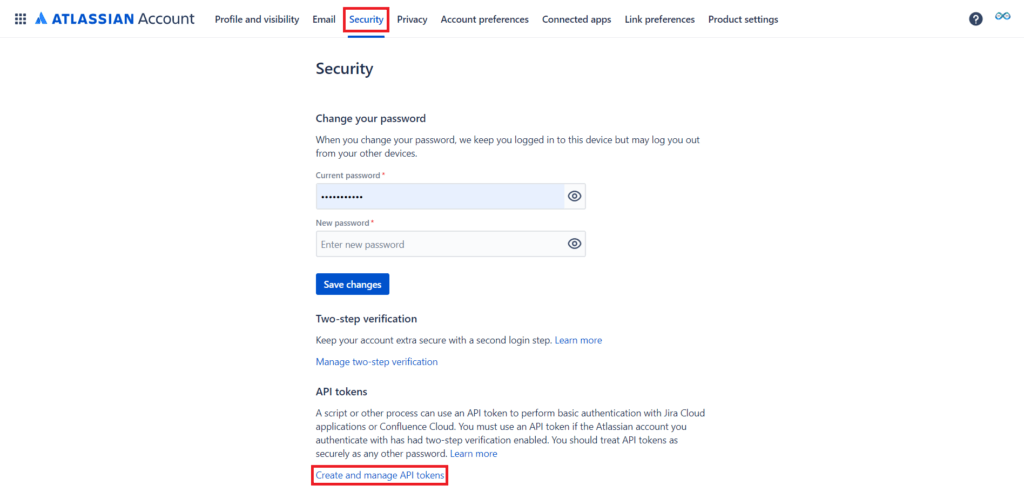
Click on Create API token.
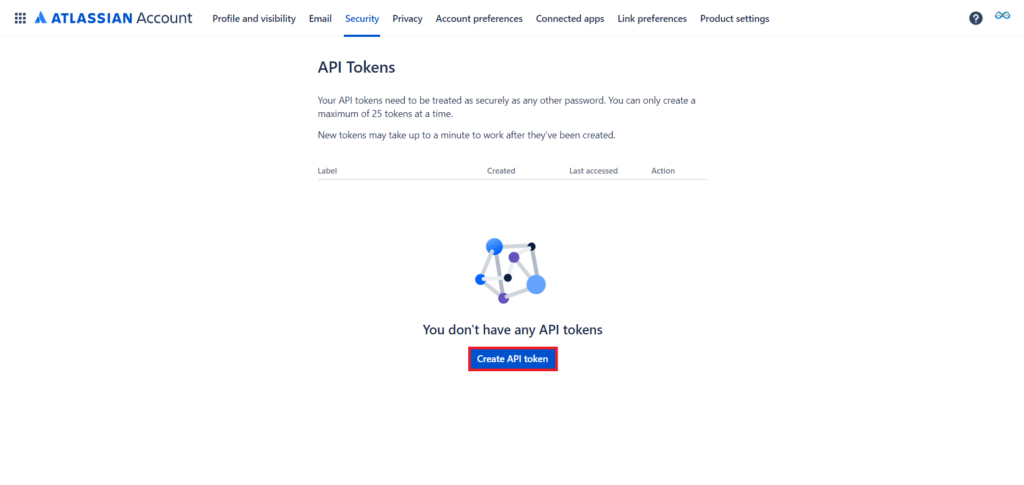
Provide a label and click on Create.
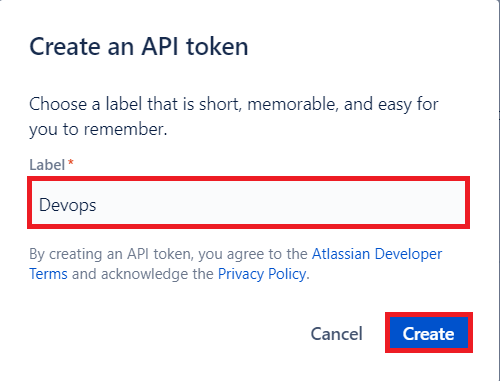
Copy the generated API token cause we will be using it later.

Now our API token is also created.

Step #3:Install Python and Required Tools
First update the system packages.
sudo apt update

Install Python, Python Virtual Environment and Pip.
sudo apt install python3 python3-venv python3-pip -y

Set up a Python Virtual Environment. A virtual environment isolates your Python environment from the global system environment, making dependency management easier and avoiding conflicts
python3 -m venv myenv

Activate the virtual environment to use it.
source myenv/bin/activate

With the virtual environment active, use Pip to install the requests library, which we need for making HTTP requests to the Jira API.
pip install Flask requests

Step #4:Write a python script
First create a directory and navigate to it.
mkdir ~/jira_integration
cd ~/jira_integration

To get the issue id to write in script go to your project>> configure board >> the issue you want to create, see url you will get issue id
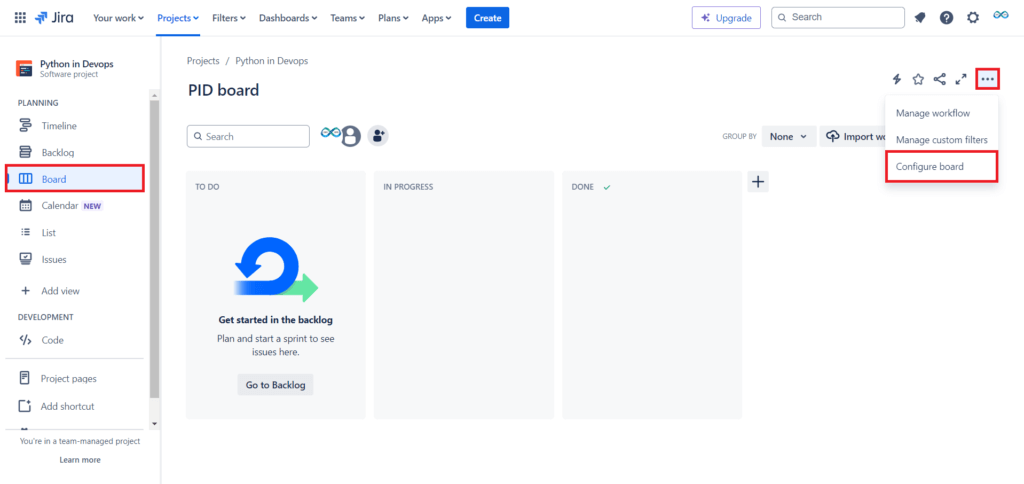

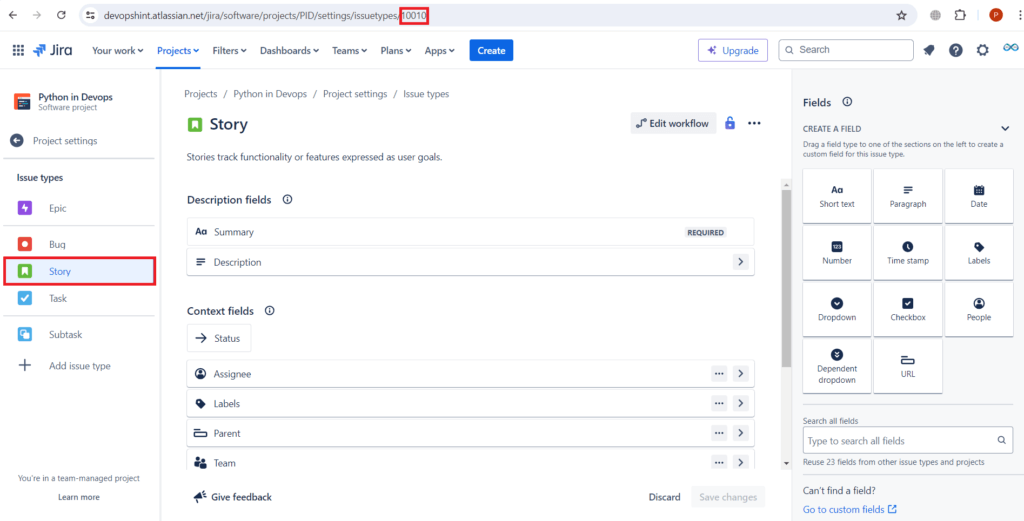
Create a file to write python script like app.py
nano app.py

Here’s a basic Python script using the requests library to handle GitHub webhook events and create Jira tickets
from flask import Flask, request
import requests
from requests.auth import HTTPBasicAuth
import json
app = Flask(__name__)
@app.route("/createjira", methods=['POST'])
def createjira():
# Jira API endpoint
url = "your_domain.atlassian.net/rest/api/3/issue"
# Authentication with Jira API
auth = HTTPBasicAuth("[email protected]", "your api token")
# HTTP headers for the request
headers = {
"Accept": "application/json",
"Content-Type": "application/json"
}
# Example payload for Jira issue creation
payload = json.dumps({
"fields": {
"description": {
"content": [
{
"content": [
{
"text": "My first Jira ticket of the day",
"type": "text"
}
],
"type": "paragraph"
}
],
"type": "doc",
"version": 1
},
"issuetype": {
"id": "your_issue_id"
},
"project": {
"key": "your_key"
},
"summary": "Main order flow broken",
},
"update": {}
})
# Get the webhook JSON data from the request
webhook = request.json
# Create a Jira issue if the comment contains '/jira'
if webhook and 'comment' in webhook and webhook['comment'].get('body') == "/jira":
response = requests.post(url, data=payload, headers=headers, auth=auth)
return json.dumps(response.json(), sort_keys=True, indent=4, separators=(",", ": "))
else:
return json.dumps({"message": "Jira issue will be created if comment includes /jira"}, sort_keys=True, indent=4, separators=(",", ": "))
# Start the Flask app
if __name__ == '__main__':
app.run(host='0.0.0.0', port=5000)

Give your own domain in url, email and API token. Also give your id and key as shown in above image. Save the file and exit.
Explanation of the Script:
- Imports
requests
: A Python library to make HTTP requests.requests.auth.HTTPBasicAuth
: For handling basic HTTP authentication.json
: To handle JSON data.flask
: A lightweight WSGI web application framework.
- Flask App Initialization
- This initializes the Flask application.
- Route Definition
- This defines a route (
/createjira
) for the Flask app that handles POST requests. When a POST request is sent to this route, thecreatejira
function is called.
- This defines a route (
- Jira API Details
- URL endpoint for creating issues in Jira. Replace
"Your_domain"
with your actual Jira domain. - Basic authentication using an email and an API token. Replace
"[email protected]"
and"API_TOKEN"
with your actual credentials.
- URL endpoint for creating issues in Jira. Replace
- Headers
- HTTP headers specifying that the request will accept and send JSON data.
- Payload Construction
- The payload is a JSON object containing the details of the Jira issue to be created. It includes:
description
: A detailed description of the issue in Atlassian Document Format (ADF).project
: The key of the Jira project where the issue will be created. Replace"Your_Key"
with your actual project key.issuetype
: The ID of the issue type. Replace"Your_ID"
with your actual issue type ID.summary
: A brief summary of the issue.
- The payload is a JSON object containing the details of the Jira issue to be created. It includes:
- Sending the Request
- This sends a POST request to the Jira API with the constructed payload, headers, and authentication.
- Returning the Response
- Converts the response from Jira to a formatted JSON string and returns it as the response from the Flask route.
- Running the Flask App
- This block ensures that the Flask app runs if this script is executed directly. The app will listen on all network interfaces (
0.0.0.0
) and on port5000
.
- This block ensures that the Flask app runs if this script is executed directly. The app will listen on all network interfaces (
Run the script.
python app.py

Step #5:Setting Up GitHub Webhook
Go to Your GitHub Repository. Navigate to the repository where you want to set up the webhook.
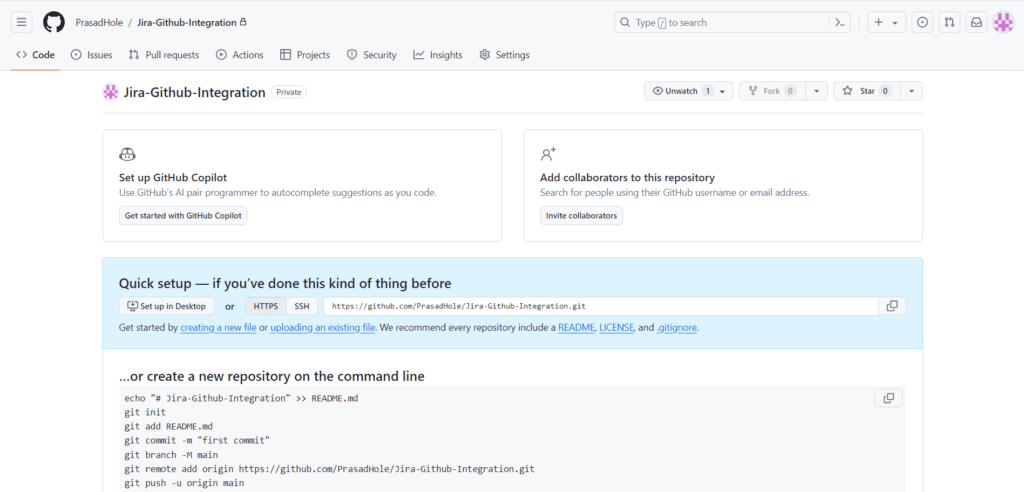
Click on Settings, then Webhooks.
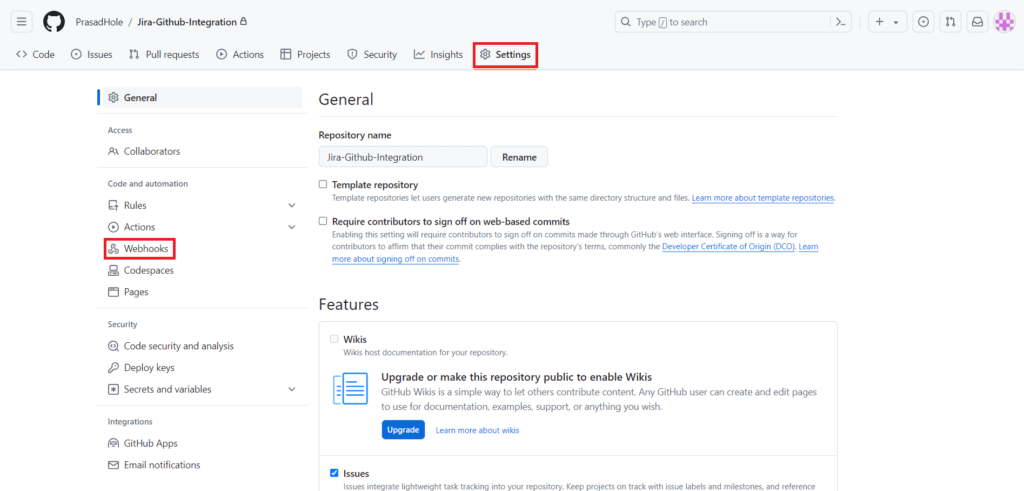
Click Add webhook.
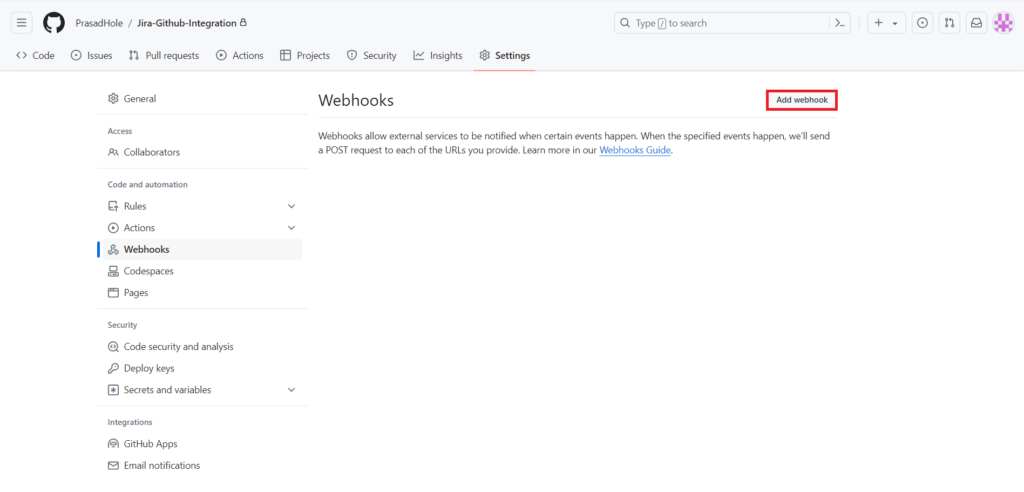
Copy the Public IPv4 DNS.

Set the Payload URL to the endpoint where your Python script will be running (e.g., http://your-public-IPv4-DNS:5000/github-webhook
).
Set the Content type to application/json.
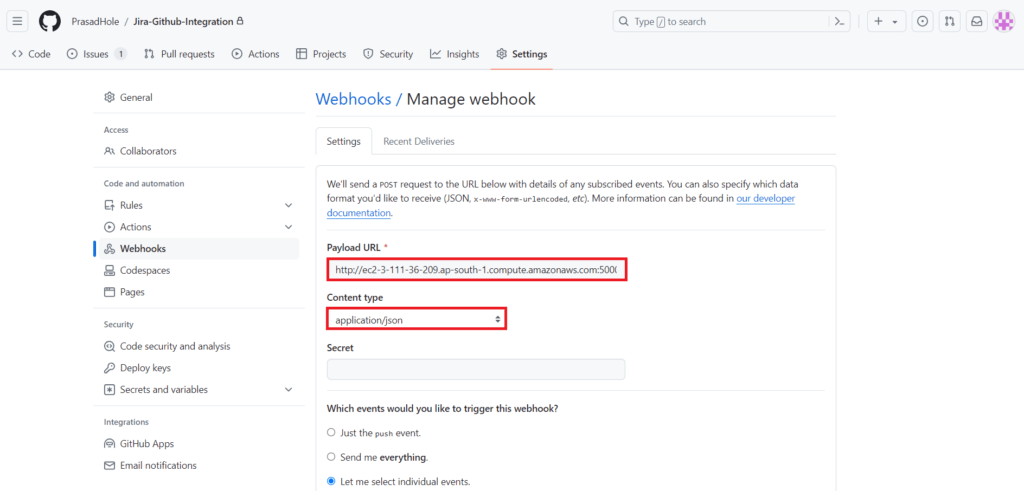
Select Let me select individual events and select the Issue comments event instead of push.
Then click on add webhook.


Now go to an issue in your GitHub repository and add a comment starting with /jira
.

Check your Jira project to see if a new ticket has been created corresponding to the GitHub comment.

Conclusion:
In conclusion, automating Jira ticket creation from GitHub comments using Python can significantly streamline the issue management process, reducing manual effort and ensuring more efficient tracking of project tasks. This integration leverages webhooks and API interactions to seamlessly connect GitHub and Jira, allowing developers to create and manage tickets directly from their development environment. By following the steps outlined in this article, you can set up this integration, enhance your DevOps workflow, and improve your team’s productivity.
Related Articles:
Python Script to Create Jira ticket
Reference: